Homework #8 |
CMSC 131
|
Due April 6th, 2003 |
Object-Oriented
Programming I |
|
Spring 2004 |
Hangman
This homework will give you experience designing (and implementing) a complete application
from start to finish.
- 70% - Hangman
- 20% - Code Style
- 10% - Time Log
You will design and implement a simple "hangman" program that allows the
player to guess a word. The idea of this game is that the computer
randomly selects one of several predefined words, and the player has to guess
it. You will use the JOptionPane.showInputDialog() and
JOptionPane.showMessageDialog() methods to get input from the player and to
display information to the player. A sample screenshot of a reasonable
solution follows:
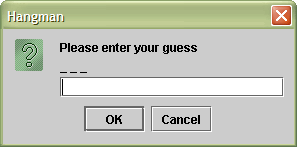
Click here to see a sequence of screenshots
of an entire game play.
Your solution must support the following features:
- Your solution must be contained within one class called Hangman that
must be contained with a package called 'hangman'.
- Randomly pick one of the words passed in to the constructor and have the
player guess that word. You may assume that at least one word is
passed in to the constructor. There is a specific signature for your
constructor that you must follow - see the 'Your Assignment' section for
more details.
- Check if the player enters a valid guess, and warn them otherwise.
A valid guess is a single character between A and Z. If they enter a
lower-case letter, that should be treated the same as an upper-case letter.
If zero or more than 1 character is entered, that should be treated as an
invalid guess. Note that the words passed in to the constructor may be
any combination of lower and upper case characters, and for this game, they
should all be treated as upper case. That is, guessing 'T' on the word
'test' should match the 't's.
- For any valid guess, warn them if they already guessed that character.
- For any valid guess where they didn't already guess that character, tell
the player if there guess resulted in any new characters being uncovered,
and if so, how many.
- After each turn, display the current guess with one underscore ('_')
character per letter of the word to be guessed for each unguessed character,
and display the actual (capital) character of each guessed character.
Display a space between each character. For example, for a three
letter word, the display should initially show "_ _ _". But if the
middle letter has been guessed as a 'A', then it should show "_ A _".
- If the player enters a valid guess that has not been guessed before,
then all characters in the word that equal that letter should be exposed.
I.e., guessing 'T' when the hidden word is "test" should display "T _ _ T".
- The game should end under either one of two conditions:
- The player correctly guesses the word, in which case, output a
congratulatory message and the number of guesses they made to win.
- The player presses 'cancel' before the he/she guesses the word. In
this case, output the number of guess they made before giving up.
- When the game ends, your final message should include only the 'OK'
button (do not display the 'Cancel' button).
Some Java note:
- You should use the JOptionPane.showInputDialog() and
JOptionPane.showMessageDialog() methods to get information from the user,
and display information to the user, respectively. You can refer to
the examples shown in class that use these methods, and available in the
code snippets on March 12 (and others), or
see the javax.swing.JOptionPane API docs for more info. Note that
JOptionPane.showInputDialog() returns null if the Cancel button is pressed.
Note that JOptionPane.showMessageDialog() displays only an 'OK' button
without a 'Cancel' button.
- You can use the Character.isLetter() method to determine if a particular
character is a letter (see java.lang.Character API docs for more info).
- You can use the String.toUpperCase() method to convert a string of
characters to all uppercase characters (see java.lang.String API docs for
more info).
- You can use integer-style operations on "char" variables.
For example, if 'c' is a variable of type char, you can check if
(c >= 'A'). You can also subtract one
character from another, which gives the distance between two characters.
For example, ('A' - 'A') == 0 and
('D' - 'A') == 3.
Getting the Code Distribution
We will be using CVS with AutoCVS as we have for previous assignments.
You can access the assignment code by checking it out from CVS with Eclipse (it
is called 'hw8'). Note that since you are writing the entire assignment,
when you checkout hw8, all you will get is a nearly empty file named
Hangman.java (containing just the package, class and constructor declaration). However, you should still check this out and edit this file
as you will use AutoCVS to submit this file back to us. You will also get
the timelog this way.
Your Assignment
You must design and implement Hangman according the above requirements.
Your Hangman class must be specified within the 'hangman' package and must
contain the following constructor where 'words' is an array of words to select
from for the player to guess. You should also have a simple main program
that runs Hangman with an array of several words. We will test your
Hangman constructor with our own set of words.
public Hangman(String[] words)
Note that you are free to write whatever code you feel is necessary to
implement this project, including instance variables, methods, and even other
classes if you feel that is the best way to create your solution.
Your code style will be graded on:
- Good choice of variable, method and class names
- Good breakdown of logic into small and clear methods, each with a
specific function. While we leave it to you to design the clearest
breakdown of your code into methods, we expect it to be unlikely that we
will consider satisfactory a solution with a single long method.
- Good spacing, line breaks, and indentation
- Good inline comments and brief method and class description comments.
Note that while you do not have to generate javadoc-style comments, you do
have to describe what each method and class does.
Submitting Your Assignment
Submit your program by right-clicking on your project and select "Submit
Project". Note that this option will only appear if you have successfully
installed AutoCVS as described previously. If you decide that you would like to
make a change after you have submitted your project, you can resubmit as many
times as you like before the extended deadline. We will grade the last project submitted.
/* Name
* Student ID * Homework #8
*/<your code goes here> |
Closed Assignment
This is a "closed" assignment. You must
do this assignment entirely by yourself. You may not look at other
people's code or show your code to others (except for the class
instructors and TAs). Any violations will be considered academic
dishonesty and will be handled as such.
Challenge Problem
Extend hangman to give the player hints. For each incorrect guess the
player makes, tell them if the nearest character in the word is less then
(closer to 'A') or greater than (closer to 'Z') the character they guessed.
Extend hangman to offer the ability to play several games simultaneously with
the same set of guesses. Start by asking the player how many games they
want to play at the same time. Then, for each character they guess, apply
that guess to all the active games, and display all the results at once.
You should write this code in a nice object-oriented fashion, so there should be
one instance of a class per game, and there should be some wrapper code that
asks the play for their guess and supplies that guess to all hangman instances.