Custom Widgets
What is a widget?
- Region on the screen with a model, view, and controller
- Controller is the part that lets users interact with view
- View and controller typically not separated
We can define custom widgets by extending UserControl, much as we've
done before
We must clearly define a public API and semantics
Goal is reusable, generic code
Must take care to avoid any connections with the rest of your
application
Example - Round Button
To create a reusable control, you need to follow these steps in
Visual Studio:
- Create a new "Blank Solution"
- In the solution explorer, right click on the solution, and
Add->New Project. Create a "Class Library" project which will create
a DLL (rather an EXE). Name this project the name of your
control (i.e. "RoundButton"). Right the code for your custom
control here. Note that to use a custom control in the forms
designer, it must have a default (i.e., no parameter) constructor.
You'll have to manually add a reference to any libraries you use.
In the References section of the project in the solution explorer,
select Add Reference. In the .NET tab, select the libraries
you want - you'll need at least System.Windows.Forms.dll and
System.Drawing.dll.
- Change the Namespace within your code of this project to be
something different than the project name (perhaps something like
your institution and name "UMD.Bederson"). There is a bug in
the forms designer that will get you later if you don't do this.
- Right click on the solution, and Add->New Project. Create
a "Windows Application" and name it something like "RoundButtonTester".
We'll use this application to test the control. Right click on
this project, and select "Set as Startup Project".
- In the form designer for your testing application, select the
"My User Controls" tab of the toolbox. Right click and select
"Add/Remove Items". Click the Browse button and select the .dll
of your new control. Your control will then show up in the
toolbox, and you can drag it onto your form.
Here is a simple example of a round button which was modified from
this example which walks you through the creation of this control.
http://www.ondotnet.com/pub/a/dotnet/2002/03/18/customcontrols.html
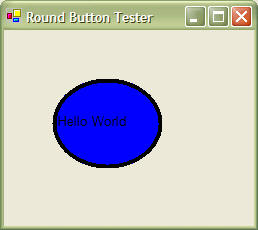
Here's the project in
RoundButton.zip
Example - Range Slider
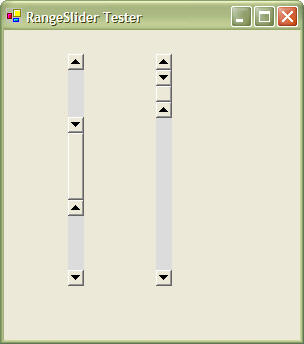
RangeSlider is a more complex example because it contains a richer
model, and interactive sub-components. This implies that the
application must know where each sub-component lies within the widget,
and how the even handlers should control them. Note that the event
handlers just updates the model, and then lets the renderer redraw the
widget appropriately.
The complete solution is in
RangeSlider.zip |